10 Tips for Optimizing React Native App Performance
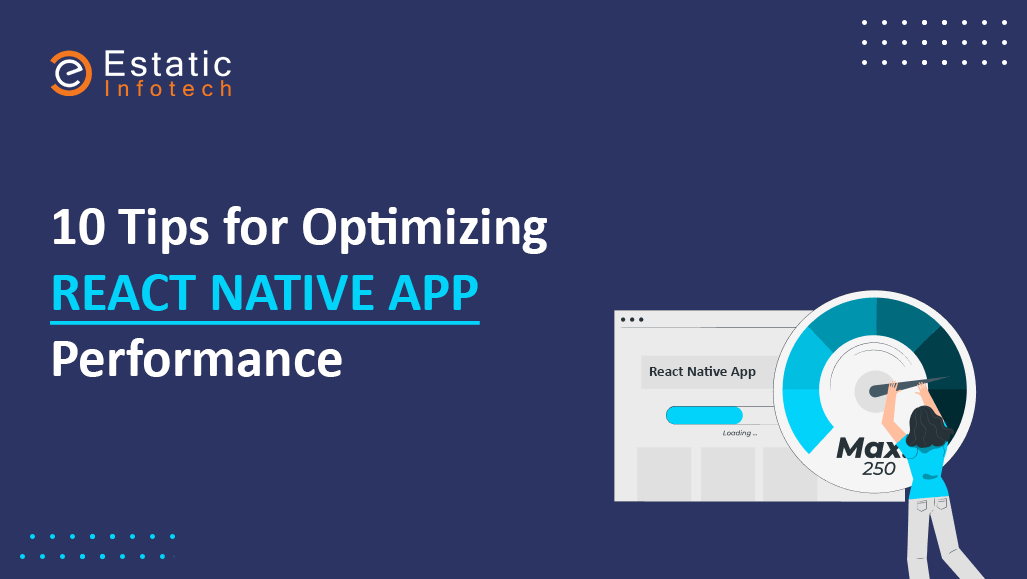
Introduction
As we all know, performance optimization stands as a pivotal concern when it comes to app development. React Native has become a top option for developers looking to quickly create reliable apps due to its promise of cross-platform efficiency. Ensuring optimal performance is always a challenge, though, especially as applications get larger and more advanced. It is imperative to pay close attention to every detail when developing a React Native application, from improving memory management to reducing rendering inefficiencies.
In this blog, we'll explore React Native performance tips and tricks to empower developers in their quest for efficiency. Whether you're embarking on a new project or seeking to enhance the performance of an existing application, these insights are poised to elevate your development endeavors.
Table of Content
10 React Native Performance Optimization Tips
Have a look at the below mentioned React Native tips that will help ensure your app is optimized for performance, providing a smooth and responsive user experience:
#1. Minimize Component Re-Renders
During the process of React Native development, over-rendering your app can slow down performance. To avoid needless re-renders, use shouldComponentUpdate, PureComponent, or React.memo. Additionally, avoid binding functions in render methods and implement appropriate key management for lists.
Memoization is handled by React.memo(), which means that rendering overhead is reduced if a component receives the same set of properties more than once. This will render the JSX view given by the functional component just once using the previously cached properties.
import React, { useMemo } from 'react';
import { View, Text } from 'react-native';
const MyComponent = ({ data }) => {
const { fields } = data;
const memoizedResult = useMemo(() => {
const processedFields = processData(fields);
const result = transformData(processedFields);
return result;
}, [fields]);
return (
<View>
<Text>Result: {memoizedResult}
</Text> </View>
);
};
export default MyComponent;
In the above example, useMemo will return the stored value if the declared dependency (data) hasn't changed after calling its calculation function() => without arguments. In the initial render; if not, it will call the calculation function with the new arguments and cache the results.
#2. Optimize Images
If you want to show off a lot of graphical content or images in your React Native app, you need optimize your images to make it run better. If the resolution and size of the rendered photos are not correctly optimized, rendering several images may result in excessive memory utilization on the device. This could overburden your app's memory and cause it to crash.
The largest assets in an app tend to be images and to maximize them:
- Make use of the proper image format and size.
- Put caching and lazy loading into practice.
- For improved performance, make use of image optimization packages such as react-native-fast-image.
import React from 'react';
import { FlatList, Text } from 'react-native';
const data = [...];
// An array of data
const VirtualizedListExample = () => {
return (
<FlatList
data={data}
keyExtractor={(item) => item.id}
renderItem={({ item }) => <Text>{item.title}
</Text>}
/> );
}
#3. Use Virtualized Lists
Use SectionList or FlatList in place of ScrollView for huge data sets. By rendering only what is currently visible on the screen, these components enhance performance and use less memory.
Also, VirtualizedLists provide props for fine-grained control over optimization configuration, like updateCellsBatchingPeriod to set the ms interval between batch renders and maxToRenderPerBatch to control the number of items rendered per batch when scrolling through the list. You may improve it even further by using FlashLists, which provide a set of additional props to further adjust performance and render stuff quickly.
#4. Use Native Modules
For resource-intensive operations like elaborate animations or large computations, use native modules. Native modules can perform better than their JavaScript equivalents since they are written in platform-specific languages like Java, Swift, and Objective-C.
#5. JavaScript Bundles Optimization
Minimize the size of your JavaScript bundle. Use programs such as Webpack or Metro bundler to divide code and load only the required portions. You should also activate tree shaking so that your bundles contain no unnecessary code.
Another important aspect that comes to mind while React Native development is speed, which can be optimized through JS Bundles. It is essential for improving user experience because it ensures faster app access and lowers initial load times. Users with data plans that are limited will also benefit from it because smaller bundles save money. JavaScript engines handle smaller bundles faster, which enhances the responsiveness and performance of apps. Also, there are frequent size restrictions for software submissions in shops like the Google Play Store and the Apple software Store. Ensuring that your app satisfies these standards means controlling the size of your bundle.
Here are a few techniques for maximizing the bundle size of your React Native application.
- Code splitting: Load only the code necessary for the active screen or feature, dividing the larger codebase of your app into smaller bundles. React Native does not by default allow code splitting, but you may take advantage of React Native's multi-bundle support, module federation, and code-splitting capabilities by using third-party tools like Re.Pack.
- Asset Optimization: Compress the fonts, images, and other materials that are used in your application. Use WebP for greater compression and SVG for resolution-independent vector graphics when creating images.
- Dependency Management: Take care while incorporating third-party libraries and dependencies into your work. Include only the necessary information, audit dependencies frequently, and upgrade to the most recent versions of any dependencies—which may have size optimizations.
- Bundle Analyzer: Visualize your application's bundle using tools such as react-native-bundle-visualizer to spot code segments that can be streamlined or huge dependencies. For example, to optimize bundle size, one can choose to forego momentjs in favor of dayjs, and the analyzer will show this.
#6. Optimize Network Requests
Reduce the number and size of requests made to the network. To handle requests more effectively, use a library such as Axios, and think about using caching techniques to lower the number of network calls. Also, use lightweight data formats like JSON rather than XML.
Here are examples demonstrating several methods for optimizing network queries with the react-query library, but numerous additional tools can accomplish the same goal.
Caching: To minimize needless network requests and improve app responsiveness while simultaneously decreasing server load, cache previously fetched data and return it for subsequent requests.
import { useQuery } from 'react-query';
const MyComponent = () => {
const { data } = useQuery('myData', fetchDataFunction, {
// Enable caching with a specific cache time (e.g., 10 minutes)
cacheTime: 1000 * 60 * 10,
});
// Your component logic here
};
Pagination: Use pagination to acquire and show data in smaller parts so that enormous data sets can be displayed more effectively. This will minimize the quantity of data held in memory, help lower the amount of data carried over the network and shorten the initial load time.
import { useInfiniteQuery, useQueryClient } from 'react-query';
const MyComponent = () => {
const queryClient = useQueryClient();
const { data, fetchNextPage, hasNextPage } = useInfiniteQuery(
'myData',
fetchDataFunction,
{
getNextPageParam: (lastPage) => lastPage.nextPageToken, // Modify this based on your API response
}
);
const loadMore = () => {
if (hasNextPage) {
fetchNextPage();
}
};
#7. Reduce UI Complexity
Reduce complexity in your user interface to improve efficiency. Steer clear of complicated layout computations and highly nested components. To speed up rendering times, use layouts that are simpler and have fewer nested layers.
#8. Avoid Unnecessary Dependencies
The bundle size and load time of your program increase with each dependency. Audit your dependencies frequently to get rid of any unwanted or unused libraries. Choose lightweight substitutes whenever it's feasible.
#9. React Native Debugger
React Native Debugger is a useful tool for performance bottleneck identification and benchmarking. Using this tool, you may locate ineffective parts and processes and implement focused adjustments.
#10. Memory Management
Optimizing memory usage is essential for a seamless user experience. By removing timers, intervals, and subscriptions in the componentWillUnmount lifecycle method, memory leaks can be prevented. To monitor and control memory utilization, use programs like Android Profiler and Xcode Instruments.
To Sum Up
With a plethora of fresh information at our disposal, it is obvious that improving the speed of React Native apps is a complex but worthwhile effort as we come to an end. Whether you are looking to hire dedicated Xamarin developers or enhance your existing team, developers may confidently negotiate the challenges of performance optimization by embracing best practices in coding and architecture, minimizing rendering overhead, and utilizing native modules sparingly.
It's crucial to create applications that are quick and responsive since user expectations are rising and technology is changing at a rapid pace. Developers are getting closer to the holy grail of flawless user experiences and increased productivity with each improvement they implement. Every enhancement, regardless of how it reduces milliseconds from offloading times or maximizes memory usage, adds to the application's overall success.
Ready to put these strategies into action? Hire React Native developers who can seamlessly implement these optimizations and take your app to the next level.
Frequently Asked Questions
Why is optimizing performance important in a React Native app?
A React Native app's performance must be optimized to provide a fluid and responsive user experience. It makes sure the application runs well on many devices and keeps user satisfaction levels high.
How does performance optimization impact user engagement and retention?
Improved performance is achieved by shorter load times, seamless transitions, and overall well-optimized RN applications. This ultimately contributes to the success of the app by increasing user satisfaction, retention rates, and engagement.
What are the consequences of poor performance in a React Native app?
Poor performance in a React Native application can lead to frequent crashes or freezes, sluggish UI responsiveness, and slow loading times. User annoyance, unfavorable reviews, and eventually a decline in users and income can result from this.
What are the long-term benefits of investing in performance optimization for a React Native app?
For a React Native app, speed optimization can result in long-term advantages including increased brand recognition, steady user growth, and less maintenance expenses. Additionally, it sets up the app for future improvements and scalability.
How can performance optimization impact the app's compatibility with various devices and platforms?
Through performance optimization, you make sure that the application functions flawlessly on many platforms and devices, irrespective of their operating systems or hardware configurations. This increases the app's accessibility and reaches a larger user base.
How do you ensure the quality of React Native apps developed by your team?
We perform thorough testing procedures, such as unit, integration, and user acceptability testing, to guarantee the caliber of React Native applications. To produce dependable, high-quality apps, we also conform to coding standards and industry best practices.
Do you provide custom React Native app development services?
Yes, we specialize in custom RN app development tailored to the unique requirements of each client. Whether you need a standalone app or integration with existing systems, we can deliver a solution that meets your specific needs.