How Can You Effectively Integrate Firebase into Your Android App?
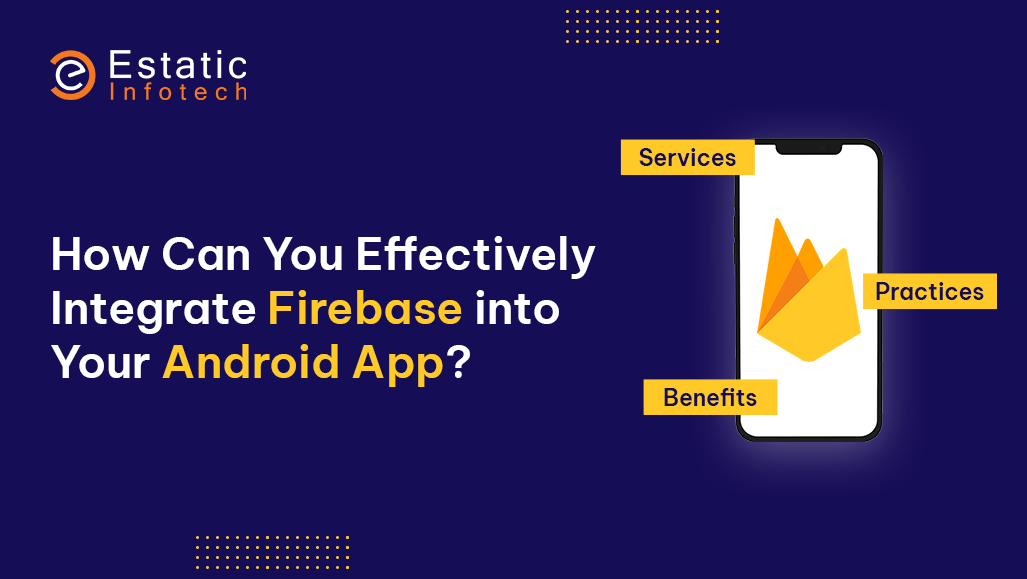
You may greatly improve your Android app's usability, scalability, and functionality by integrating Firebase into it. Google's Firebase platform, which comes with a range of tools and Android app development services, makes development easier and gives strong back-end functionality without requiring a lot of infrastructure. We'll go over the procedures and best practices for successfully integrating Firebase into your Android app in this article.
Understanding Firebase and Its Benefits
The extensive Firebase platform offers a wide range of services, including analytics, authentication, real-time databases, cloud messaging, and more. By utilizing Firebase, developers may concentrate more on creating interesting app features and less on maintaining server infrastructure. The following are the main advantages of including Firebase in your Android app:
- Real-time Data Sync: By enabling you to store and synchronize data in real-time across all clients, Firebase Realtime Database offers a smooth user experience.
- Scalable Hosting Solutions: To guarantee that your app can expand, Firebase provides scalable hosting for static files and dynamic content.
- Sturdy Authentication: Firebase Authentication streamlines user authentication by enabling social media, email, and password logins, among other login methods.
- All-Inclusive Analytics: Firebase Analytics helps you make data-driven decisions to enhance your app by offering insights into user behavior.
- Push Notifications: To engage and keep users, you can deliver customized notifications using Firebase Cloud Messaging (FCM).
- Machine Learning Capabilities: You don't need to be an expert in machine learning to incorporate its capabilities into your app with Firebase ML Kit.
You Can Also Refer: "Add Firebase to your Android project"
Setting Up Firebase in Your Android App
To get started with Firebase, follow these steps to integrate it into your Android project:
Step 1: Create a Firebase Project
Go to the Firebase Console: Visit Firebase Console and sign in with your Google account.
Create a New Project: Click on "Add Project" and enter a project name. Optionally, enable Google Analytics for your project.
Add Android App: Once your project is created, click on "Add App" and select the Android icon. Enter your app's package name and register it.
Step 2: Add Firebase SDK to Your App
Download the google-services.json File
After registering your app, download the google-services.json file and place it in the app/ directory of your Android project.
Update Project-level build.gradle
Add the Google services classpath to the dependencies section.
buildscript {
dependencies {
classpath 'com.google.gms:google-services:4.3.3'
}
}
Update App-level build.gradle
Apply the Google services plugin and add Firebase SDK dependencies.
apply plugin: 'com.android.application'
apply plugin: 'com.google.gms.google-services'
dependencies {
// Firebase SDKs
implementation 'com.google.firebase:firebase-analytics:18.0.2'
}
Also Read: How Much Does Android App Development Cost
Step 3: Initialize Firebase in Your App
Initialize Firebase: In your app’s Application class or MainActivity, initialize Firebase using the FirebaseApp.initializeApp(this) method.
import com.google.firebase.FirebaseApp;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
FirebaseApp.initializeApp(this);
setContentView(R.layout.activity_main);
}
}
Integrating Firebase Services
After Firebase is configured, you can begin incorporating its features into your application. Here are some tips for utilizing some of the main Firebase services:
1. Authentication with Firebase
Firebase Authentication streamlines the user authentication process by offering several sign-in options, such as social media logins, phone numbers, email addresses, and passwords.
- Email/Password Authentication
To enable email/password authentication, go to the Firebase Console, navigate to the Authentication section, and enable the Email/Password provider.
FirebaseAuth mAuth = FirebaseAuth.getInstance();
// Sign up a new user
mAuth.createUserWithEmailAndPassword(email, password)
.addOnCompleteListener(this, task -> {
if (task.isSuccessful()) {
// Sign-up success
} else {
// If sign-up fails, display a message to the user.
}
});
- Google Sign-In
To integrate Google Sign-In, enable the Google provider in the Firebase Console and configure your app to use GoogleSignInOptions.
GoogleSignInOptions gso = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestIdToken(getString(R.string.default_web_client_id))
.requestEmail()
.build();
Read More: Questions You Should Ask Before Choosing Android App Development Company
2. Firebase Realtime Database
Firebase Realtime Database allows you to store and sync data across your users in real-time. To use it:
- Enable Realtime Database
In the Firebase Console, navigate to the Realtime Database section and create a database.
- Read and Write Data:
DatabaseReference database = FirebaseDatabase.getInstance().getReference();
// Write data
database.child("message").setValue("Hello, World!");
// Read data
database.child("message").addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String message = dataSnapshot.getValue(String.class);
// Update UI with message
}
@Override
public void onCancelled(DatabaseError databaseError) {
// Handle possible errors.
}
});
Dive In: "Security Best Practices: Building Reliable & Trusted Android Apps"
3. Firebase Cloud Messaging (FCM)
Firebase Cloud Messaging (FCM) allows you to send push notifications to users to keep them engaged.
- Configure FCM
In the Firebase Console, navigate to the Cloud Messaging section to set up notifications.
- Send Notifications
Use the Firebase Console or server-side scripts to send notifications.
FirebaseMessaging.getInstance().subscribeToTopic("news")
.addOnCompleteListener(task -> {
String msg = "Subscribed to news topic";
if (!task.isSuccessful()) {
msg = "Subscription failed";
}
Log.d("FCM", msg);
});
4. Firebase Analytics
Firebase Analytics helps you understand user behavior by tracking user interactions and events.
- Log Events
Use the Firebase Analytics SDK to log events and track user engagement.
FirebaseAnalytics mFirebaseAnalytics = FirebaseAnalytics.getInstance(this);
// Log an event
Bundle bundle = new Bundle();
bundle.putString(FirebaseAnalytics.Param.ITEM_ID, "id");
bundle.putString(FirebaseAnalytics.Param.ITEM_NAME, "name");
mFirebaseAnalytics.logEvent(FirebaseAnalytics.Event.SELECT_CONTENT, bundle);
- View Analytics
Analyze user behavior and app performance using the Firebase Console's analytics dashboard.
Best Practices for Firebase Integration
To ensure a smooth integration of Firebase services into your Android app, consider the following best practices:
1. Optimize Data Usage
Minimize data usage by using appropriate data retrieval methods, such as addListenerForSingleValueEvent for one-time data reads.
2. Secure Your Database
Implement Firebase Realtime Database security rules to restrict unauthorized access and protect user data.
3. Handle Authentication Errors
Provide clear error messages and handle authentication errors gracefully to enhance user experience.
4. Test Notifications
Regularly test push notifications to ensure they are delivered as expected and engage users effectively.
5. Monitor Analytics
Use Firebase Analytics to monitor user engagement and make informed decisions to improve your app.
6. Stay Updated
Keep your Firebase SDKs up to date to benefit from the latest features and security enhancements.
Conclusion
Integrating Firebase into your Android app offers numerous benefits, from real-time data synchronization and robust authentication to push notifications and analytics. By following the steps outlined in this guide and adhering to best practices, you can effectively leverage Firebase to enhance your app’s functionality and user experience. Whether you're building a simple app or a complex one, Firebase provides the tools and services you need to succeed.
Frequently Asked Questions
1. What is Firebase, and why should I use it in my Android app?
It is a mobile development platform by Google that offers a variety of tools and services, including real-time databases, authentication, analytics, and cloud messaging. It simplifies backend development, improves app performance, and helps you focus on building features.
2. How do I start integrating Firebase into my Android app?
Start by creating a Firebase project in the Firebase Console. Then, add your Android app by registering its package name, downloading the google-services.json file, and adding it to your project. Finally, update your app's build.gradle files with Firebase dependencies.
3. What Firebase services can I integrate into my Android app?
You can integrate various Firebase services, such as Firebase Realtime Database, Firestore, Firebase Authentication, Firebase Cloud Messaging, Firebase Analytics, and Firebase Crashlytics, depending on your app's needs.
4. How do I implement Firebase Authentication in my app?
Add Firebase Authentication dependency to your project, configure sign-in methods (e.g., email/password, Google, Facebook) in the Firebase Console, and use the Firebase Auth SDK in your app to handle user authentication and manage user sessions.
5. How can I use Firebase Realtime Database or Firestore for data storage?
First, add the necessary Firebase dependencies to your project. Then, structure your data in the Firebase Console. Use Firebase's SDK to read, write, and listen to data changes in your app, ensuring data is synchronized in real-time across users.
6. How do I set up Firebase Cloud Messaging (FCM) for push notifications?
Add Firebase Cloud Messaging dependencies to your project, configure FCM in the Firebase Console, and use the FCM SDK in your app to send and receive push notifications. You can also segment users and schedule notifications based on specific triggers.
7. How can Firebase Analytics help in tracking user behavior in my app?
Firebase Analytics automatically tracks basic user interactions and events. You can log custom events and parameters to gain deeper insights into user behavior, which can help you make data-driven decisions to improve your app.
8. What steps should I take to ensure Firebase is securely integrated into my app?
Use Firebase Authentication for secure user sign-in, enforce Firebase Security Rules for databases, and enable data encryption. Regularly review your Firebase project's security settings and monitor access through the Firebase Console.
9. Can I integrate Firebase with other third-party services in my Android app?
Yes, Firebase can be integrated with various third-party services through Firebase Extensions, Cloud Functions, and REST APIs. This allows you to extend your app’s functionality by connecting to other tools and platforms.
10. How do I monitor and optimize my Firebase-integrated Android app's performance?
Use Firebase Performance Monitoring to track app performance metrics, such as app startup time, network requests, and screen rendering. Analyze this data to identify bottlenecks and optimize your app for a better user experience.